Building a Carousel in Sitecore MVC
Part 2: Controller Renderings
Introduction
In this section we will work through building our carousel using a controller rendering. Sitecore MVC controller renderings work in the same way as in vanilla ASP.NET MVC, so if you are familiar with MVC, but new to Sitecore MVC this will feel most familiar to you.
Part 1 of this tutorial series gives an overview of the HTML markup and our Sitecore content structure, so take a look there if you need the background information.
Model(s)
Our CarouselViewModel
class will contain the fields needed for the carousel and it’s slide items, which will be represented by IList<CarouselSlideViewModel>
. Using IList
instead of IEnumerable
allows us to use a foreach
loop or a for
loop. The latter is useful for applying specific classes to each slide based on index (if you can't use CSS 3 selectors).
CarouselViewModel class definition:
public class CarouselViewModel
{
public IList CarouselSlides { get; set; }
}
CarouselSlideViewModel class definition
public class CarouselSlideViewModel
{
public Item Item { get; set; }
public MvcHtmlString Header
{
get { return GetFieldValue("Header"); }
}
public MvcHtmlString SubHead
{
get { return GetFieldValue("SubHead"); }
}
public MvcHtmlString Background
{
get { return GetFieldValue("Background"); }
}
public MvcHtmlString CallToAction
{
get { return GetFieldValue("CallToAction"); }
}
private MvcHtmlString GetFieldValue(string fieldName)
{
return new MvcHtmlString(Sitecore.Web.UI.WebControls.FieldRenderer.Render(Item, fieldName));
}
}
A note about model terminology
The term "ViewModels" is used in this section for two reasons:
- To differentiate them from
RenderingModel
s covered in part 1 (though RenderingModels are just a special type of ViewModel). - To make it clear that these classes are specific to a view and are not "Models" in the traditional MVC sense of the word.
View
The View references the CarouselViewModel
class and contains the outer markup (just a div) for the carousel, plus a foreach loop to generate the html for the carousel-slide divs.
@model SitecoreMvcCarousel.Models.CarouselViewModel
<div class="carousel">
@foreach (var carouselSlide in Model.CarouselSlides)
{
<div class="carousel-slide">
<h1>@carouselSlide.Header</h1>
<p>@carouselSlide.SubHead</p>
@carouselSlide.Background
@carouselSlide.CallToAction
</div>
}
</div>
Controller
Our controller handles the request for the carousel, creates an instance of the CarouselViewModel
class and returns a ViewResult
.
public class CarouselController : Controller
{
[HttpGet]
public ViewResult Index()
{
var item = Sitecore.Mvc.Presentation.RenderingContext.Current.Rendering.Item;
var slideIds = Sitecore.Data.ID.ParseArray(item["SelectedItems"]);
var viewModel = new CarouselViewModel
{
CarouselSlides =
slideIds.Select(i =>
new CarouselSlideViewModel
{
Item = item.Database.GetItem(i)
}).ToList()
};
return View("~/Views/Renderings/Carousel/Carousel_(ControllerRendering).cshtml", viewModel);
}
}
Controller rendering definition item
The final step is to create a controller rendering item in Sitecore (this is what you will select in your pages' presentation details).
- Under your renderings folder create a new item called “Carousel” using the ‘Controller rendering’ template
- Set the Controller field to "Carousel" (the same as your controller class name minus the word 'Controller')
- Set the Controller Action field to "Index" (the name of your ActionResult method)
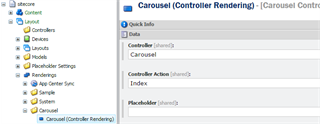
Using the controller rendering
- Go to the "Home" item, select presentation details and add the "Carousel (Controller Rendering)" rendering
- Set the datasource to point to the "Carousel" item beneath the page
Summary
In this second of three posts I have covered how to build a carousel using a Sitecore MVC controller rendering.
Reasons for choosing a controller rendering in Sitecore MVC could include:
- Unit-testability (though I would discourage actually unit testing Sitecore content)
- Building components in data-driven sites where data is displayed from sources other than Sitecore
- Performing complex item selection/filtering/ordering logic on sub-items
The complete code can be found on GitHub and if you're interested to learn more, please take a look part 3 which covers item renderings.