Building a Carousel in Sitecore MVC
Part 1: View Renderings
Introduction
In this three part tutorial I describe how to build a carousel in Sitecore MVC using view renderings (this part), controller renderings and finally item renderings. It doesn’t discuss the CSS or JavaScript aspects of the carousel, just the basic HTML markup and the necessary Sitecore templates, items, Razor and C# code.
HTML Markup
Our HTML markup is shown in the image below. Note that we may have as many carousel-slide items as we want.
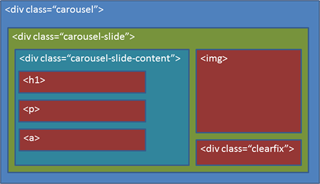
Sitecore Content for the Carousel
For this tutorial we will be focusing on the rendering and code aspects of the carousel, but as a brief overview we will use the following content structure:
- One item to define the datasource of our carousel. This is a sub-item of the page.
- One sub-item per carousel slide.
This carousel item sits beneath the page on which it is used, but there is no reason you couldn’t place it in a shared content folder outside of the main site tree, if you want to share the carousel content between multiple pages.
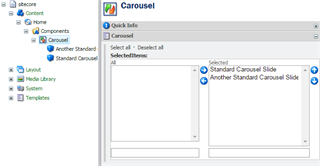
Note that the carousel item has a "SelectedItems" field where the required slides can be chosen. We could just make the assumption of using the child items of the carousel item, but this gives us a bit more flexibility and allows us to remove slides from display without deleting them.
Below is a screenshot showing the fields used on our carousel slide items.
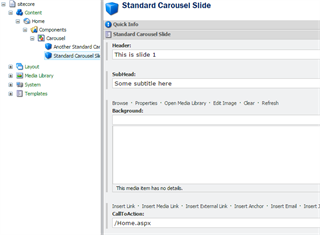
Part 1.1: Building a carousel with a Sitecore MVC View Rendering without C# code
This section describes how to build the carousel without writing any C# controller, model or rendering model code. This is a great option if you are a front-end developer, or are transitioning from XSLT renderings to MVC.
This is the approach I would also recommend for structural and container-style renderings where the intention is to provide HTML scaffolding and placeholders for other components. Note that this solution just selects the carousel slides based on the children of the datasource.
View (cshtml)
The code for the view is actually very simple. You don’t even need to include the @model directive, but it can be useful to make it clear what model the view uses. Sitecore automatically passes an instance of the Sitecore.Mvc.Presentation.RenderingModel
class to the view, so you can access two main properties of interest: Item
and PageItem
. Item refers to the datasource item set for the view in the rendering component properties, whereas PageItem refers to the context item of the page.
<div class="carousel">
@foreach (var carouselSlide in Model.Item.Children)
{
<div class="carousel-slide">
<div class="carousel-slide-content">
<h1>@Html.Sitecore().Field("Header", carouselSlide)</h1>
<p>@Html.Sitecore().Field("SubHead", carouselSlide)</p>
@Html.Sitecore().Field("CallToAction", carouselSlide)
</div>
@Html.Sitecore().Field("Background", carouselSlide)
<div class="clearfix"></div>
</div>
}
</div>
View rendering definition item
The rendering definition item is configured as shown below with the “Path” field set to our view - note that because our view rendering uses the default Sitecore RenderingModel there is no need to set the Model field.
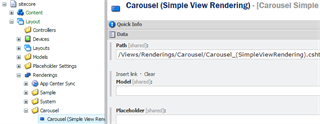
Using the View Rendering
- Go to the "Home" item and select the presentation details and add the new
Carousel (Simple View Rendering)
rendering. - Set the datasource to point to the “Carousel” item beneath the page.
Part 1.2: Building a carousel with a Sitecore MVC View Rendering and a RenderingModel
In this section we build the carousel using a Sitecore MVC View rendering with a RenderingModel. As a minimum our model must implement the Sitecore.Mvc.Presentation.IRenderingModel
interface - you can also make it inherit from Sitecore.Mvc.Presentation.RenderingModel
. I refer to this type of class as a "RenderingModel" to make the distinction from the various other types of model you could encounter in ASP.NET MVC.
You may wonder how this model is initialsed if we don’t create a controller? This is handled by the standard Sitecore controller. You will also need to create a model definition item in Sitecore which tells the Sitecore controller what class to instantiate when invoking the view rendering.
RenderingModel class
This is a simple class inheriting from Sitecore.Mvc.Presentation.RenderingModel
(or implementing the interface Sitecore.Mvc.Presentation.IRenderingModel
) which overrides the Initialize method.
public class CarouselRenderingModel : Sitecore.Mvc.Presentation.RenderingModel
{
public override void Initialize(Rendering rendering)
{
base.Initialize(rendering);
CarouselSlides =
Sitecore.Data.ID.ParseArray(Item["SelectedItems"])
.Select(id => Item.Database.GetItem(id)).ToList();
}
public IList- CarouselSlides { get; private set; }
}
View (cshtml)
The razor markup for our View Rendering is almost identical to that of the previous example, but includes the @model directive and references our CarouselSlides property instead of the child items.
@model SitecoreMvcCarousel.Models.CarouselRenderingModel
<div class="carousel">
@foreach (var carouselSlide in Model.CarouselSlides)
{
<div class="carousel-slide">
<div class="carousel-slide-content">
<h1>@Html.Sitecore().Field("Header", carouselSlide)</h1>
<p>@Html.Sitecore().Field("SubHead", carouselSlide)</p>
@Html.Sitecore().Field("CallToAction", carouselSlide)
</div>
@Html.Sitecore().Field("Background", carouselSlide)
<div class="clearfix"></div>
</div>
}
</div>
Model definition item
Under /sitecore/layout/Models
create a new Model. In the Model Type
field enter the fully qualified namespace and class name and assembly in the format: MyNamespace.MyRenderingModel, MyAssembly
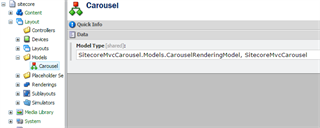
Creating the View Rendering definition item
- Under your renderings folder create a new view rendering
- In the “Path” field enter the path to the cshtml view (using forward slashes)
- In the “Model” field select the model definition item created previously
- Go to the “CarouselDemo” item and select the presentation details and add the new Carousel (View Rendering) rendering.
- Set the datasource to point to the “Carousel” item beneath the page.
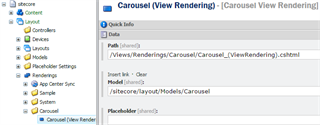
Using the View Rendering
- Go to the "Home" item and select the presentation details and add the new
Carousel (View Rendering)
rendering. - Set the datasource to point to the “Carousel” item beneath the page.
The finished carousel should now appear as shown below:
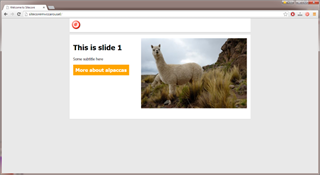
Summary
In this first post, I have covered two ways to build Sitecore MVC View renderings:
- Without C# code or rendering model - useful for front-end developers, building simple or scaffolding-like components, banishing XSLT!
- With a RenderingModel class and Model definition item - useful when you want to apply some logic/control to your item selection
The source code for the tutorial series can be found on GitHub. When you're ready please check out the next two posts on: