Building a Carousel with Personalization in Sitecore MVC
Introduction
This article describes how to build a carousel in Sitecore MVC which can be personalized. Martin English commented on a previous post inspiring me to write this, thank you Martin! I chose View Renderings but Controller Renderings or Sublayouts would also work. This solution enables the first slide(s) to use personalization and the remaining slides to use a default collection.
DataSource Content Structure
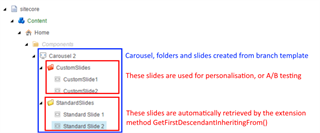
This may look complex, but a branch template makes creating a new carousel simple. The hierarchy allows the standard slides to be automatically queried making life easier for content editors.
Carousel SubTree Placement
The carousel item and subitems are beneath the page in the "Components" folder, if your carousel will be shared between multiple pages, you could put it in a “SharedContent” folder as a sibling of the Home item instead.
View Renderings for the Carousel
Our carousel is implemented with two separate view renderings.
Carousel.cshtml
This generates the containing div and the prev/next buttons for the carousel.
@using Web.Extensions
@using Sitecore.Data.Items
@using System.Linq
@model Sitecore.Mvc.Presentation.RenderingModel
<div class="carousel">
@Html.Sitecore().Placeholder("carousel-custom-slides")
@{
var folderItem =
Model.Item.GetFirstDescendantInheritingFrom(
Web.TemplateIds.StandardCarouselSlideFolder);
var slides = folderItem != null
? folderItem.Children.Cast<Item>()
: new List<Item>();
}
@foreach (var slide in slides)
{
@Html.Sitecore().Rendering(
Web.RenderingIds.CarouselSlide.ToString(),
new {Datasource=slide.ID})
}
<span class="prev">
<span class="arrow"></span>
</span>
<span class="next">
<span class="arrow"></span>
</span>
</div>
The placeholder carousel-custom-slides
is for personalized slides. It can be left empty if personalization is not required.
After the placeholder the extension method GetFirstDescendantInheritingFrom
is called. This gets the first descendant of the datasource inheriting the specified template. Using separate templates for the CustomSlides and GenericSlides folders allows us to do this.
public static Item GetFirstDescendantInheritingFrom(this Item item, ID templateId)
{
string query = string.Format(".//*[@@templateid='{0}']", templateId);
return item.Axes.SelectSingleItem(query);
}
Finally a foreach loop renders the children of the GenericSlides folder item using the Html.Sitecore().Rendering()
extension method. The variable Web.RenderingIds.CarouselSlide
is the ID of the CarouselSlide view rendering definition item in Sitecore. I always create static readonly variables for Sitecore IDs such as this, to avoid the use of magic strings.
CarouselSlide.cshtml
This outputs an individual slide. It's used in the carousel-custom-slides
placeholder and the the foreach loop of Carousel.cshtml.
@model Sitecore.Mvc.Presentation.RenderingModel
<div class="carousel-slide">
<div class="carousel-slide-content">
<h1>@Html.Sitecore().Field("Header")</h1>
<p>@Html.Sitecore().Field("SubHead")</p>
@Html.Sitecore().Field("CallToAction")
</div>
@Html.Sitecore().Field("Background")
<div class="clearfix"></div>
</div>
Configuring the Carousel
Add the carousel to a page, set the datasource to a Carousel subtree. The standard slides are automatically selected and rendered. The carousel is now functional minus personalization.
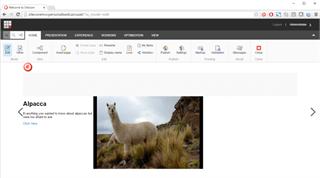
Adding a Slide with personalization
- In the experience editor select the
carousel-custom-slides
placeholder above the current slide. - Click "Add here" and select the
Carousel Slide
rendering. - Set the
Carousel Slide
datasource to an item in theCustomSlides
folder, click OK then Save. Use the arrows on the carousel to view the different slides. - With the
Carousel Slide
selected, click the the personalise button. - In the Personalise Component dialog, click
New Condition
- Enter a name for the condition.
- Edit the condition. I set up a simple condition to check if the username of the current user is admin - not a common scenario, but easy to test!
- Under Personalize Content, click [...] to select an alternative datasource item for the condition, then click OK and Save.
You should now have a working and personalized carousel! To test, log out of the experience editor and view the page. If you are logged out you will get the default slide which you set as the datasource when first adding the CarouselSlide rendering. If you log in to Sitecore as admin in a separate tab and refresh the page, you will then see the alternative slide.
Source Code
The source code is here github.com/dresser/SitecoreMvcPersonalisedCarousel. It was built with Sitecore 8.1 (rev. 160519) but should work with any version of Sitecore supporting MVC.